Table of Contents
Related Blogs
Importance of Understanding Client-Side Security
When considering where to spend limited security resources best, remember that the client side of your application is often the most exposed—and most targeted—component. Whether it’s a web browser, mobile app, or progressive web app (PWA), client-side code is fully accessible to users and, unfortunately, to attackers as well. Unlike server-side security, which occurs in a controlled environment, client-side security defends what’s running in the wild outside your organization’s perimeter. As a result, attackers have ample opportunities to reverse-engineer, manipulate, or inject malicious scripts into your applications. Understanding and addressing client-side security risks is critical to safeguarding sensitive user data, ensuring application integrity, and maintaining customer trust in a world where threats are constantly evolving. Let’s dive in:
Overview of Common Client-Side Threats
Client-side security threats take many forms, each with unique methods for exploiting vulnerabilities. Attackers can use:
Cross-Site Scripting (XSS) | Cross-Site Request Forgery (CSRF) | Clickjacking | Man-in-the-Middle (MitM) Attacks |
---|---|---|---|
Injects malicious code into web pages, allowing attackers to steal data or hijack user sessions. | Tricks users into performing unintended actions on your application without their knowledge. | Hides malicious content beneath legitimate elements, deceiving users into interacting with the wrong interface. | Intercepts communication between users and your application, allowing attackers to eavesdrop or manipulate data in transit. |
Malicious browser extensions, insecure client-side storage, compromised third-party scripts, and phishing schemes further compound the risks, threatening both your users and your brand’s integrity. In this article, we’ll explore these common threats and provide actionable strategies to mitigate each, helping you prevent your client-side applications from becoming threat vectors.
Cross-Site Scripting (XSS)
Definition and Types of XSS
Reflected XSS
Reflected Cross-Site Scripting (XSS) occurs when an attacker injects malicious scripts into a web request, which are then reflected off a web server and executed in the user’s browser. Unlike other forms of XSS, reflected XSS relies on tricking users into clicking a specially crafted link or submitting a malicious form. Once executed in the victim’s browser, the malicious script can steal sensitive information, hijack user sessions, or even perform actions on behalf of the user. Reflected XSS is often in search fields, form submissions, or URL parameters that echo back user input without proper validation or encoding. The transient nature of reflected XSS makes it dangerous yet harder to detect, as attackers do not permanently store the malicious script on the server.
Stored XSS
Stored Cross-Site Scripting (XSS), also known as persistent XSS, is one of the most dangerous forms of XSS because the malicious script is permanently stored on the server and delivered to users each time they visit the affected page. Attackers exploit input fields, such as comment sections, message boards, or user profile pages, to inject malicious code that gets saved in the application’s database. When unsuspecting users load the compromised page, the malicious script executes in their browser without requiring any interaction, such as clicking a link. Stored XSS can be especially damaging, as it has the potential to affect many users over an extended period. This type of attack can lead to stolen credentials, unauthorized actions, and data theft, all while users remain unaware that attackers have targeted them.
DOM-Based XSS
DOM-Based Cross-Site Scripting (XSS) occurs entirely within the client-side environment, making it a purely client-side vulnerability. In this type of attack, the malicious script is injected into the Document Object Model (DOM) of a web page, manipulating the way the browser interprets and displays the page without any server interaction. The attacker typically exploits client-side JavaScript that dynamically updates the web page based on user input or URL parameters. Since the payload never reaches the server, traditional server-side protections such as input validation may not prevent it. DOM-based XSS is particularly dangerous because it leverages trusted scripts running in the browser to execute harmful actions, such as stealing sensitive information or hijacking user sessions. Effective prevention requires careful handling of user input on the client side, along with security measures like secure JavaScript coding practices and proper validation within the DOM itself.
Real-World Examples of XSS Attacks
Cross-Site Scripting (XSS) has been a prominent attack vector in numerous high-profile security incidents. Notable examples include:
- MySpace “Samy Worm” (2005): An attacker exploited a Stored XSS vulnerability on MySpace, embedding malicious code into a user’s profile. Every visitor to the compromised profile unknowingly executed the script, spreading the attack to other profiles and creating a self-replicating worm known as the “Samy Worm.”
- eBay Reflected XXS (2014): Attackers injected malicious scripts into the site’s search bar, which led to phishing pages disguised as legitimate eBay content.
- Facebook DOM-based XXS (2020): The DOM-based vuln was a particularly egregious weakness in Facebook’s payments redirect page and allowed attackers to gain control of end-users’ accounts.
Together, these incidents demonstrate the widespread impact of XSS.
Prevention and Mitigation Strategies for XSS
Input Validation
Input validation is a fundamental defense against Cross-Site Scripting (XSS) attacks. You can significantly reduce the risk of injecting malicious scripts by ensuring that all user inputs are properly sanitized and conform to expected formats before the application processes them. Input validation should be implemented both on the client and server sides, using whitelisting techniques, allowing only known safe characters and data types. Rejecting or escaping unexpected characters such as `<, “>,` `&,` or quotation marks helps prevent malicious code from being executed in the browser. However, input validation alone is not a silver bullet; it should be combined with other defensive strategies, such as code obfuscation and Content Security Policy, to form a more comprehensive XSS mitigation approach.
Output Encoding
While input validation ensures that malicious data never enters your application, output encoding protects against any harmful scripts that make it through by safely rendering user-provided data in the browser. Output encoding transforms potentially dangerous characters into a format the browser interprets as plain text rather than executable code. For example, characters like `<, “>,` and `”` are converted into their HTML entity equivalents:
`<,` `>,` and `",`
This ensures that even if an attacker manages to inject malicious code into your application, the browser will display it as harmless text instead of executing it. Output encoding should be applied consistently to all user-generated content and data displayed dynamically in HTML, JavaScript, or CSS. With input validation, output encoding forms a crucial defense mechanism against XSS attacks by ensuring that even injected code cannot be executed.
Content Security Policy (CSP)
Content Security Policy (CSP) is an advanced security mechanism that helps prevent XSS attacks by controlling which resources a browser can load and execute on a web page. By defining a strict set of rules in HTTP headers, CSP enables you to specify trusted sources for scripts, styles, and other content, blocking unauthorized scripts from running. For instance, you can limit the execution of JavaScript to files hosted on your domain and disallow inline scripts or scripts from untrusted third-party sources. CSP also allows you to block unsafe practices, like the use of `eval(),` which can lead to code injection vulnerabilities. When implemented correctly, CSP is a robust safeguard that prevents attackers from loading or executing malicious code, even if it’s been injected. While CSP isn’t a standalone defense, it complements code obfuscation, input validation, and output encoding by providing an additional barrier against XSS attacks.
Application Hardening (Obfuscation and Anti-Tamper)
While traditional defenses like input validation and Content Security Policy (CSP) play crucial roles in preventing XSS attacks, application hardening—through techniques like obfuscation and anti-tamper—offers an additional layer of protection, especially for client-side code. Obfuscation makes your JavaScript code more difficult for attackers to understand or reverse-engineer, reducing the chances of an attacker successfully injecting or exploiting malicious scripts. Anti-tamper measures help detect and prevent unauthorized changes to your code at runtime, alerting you to potential attacks. These techniques make it harder for attackers to modify or manipulate your application’s logic, even if they have initiated an XSS attack. Application hardening can make exploitation much more difficult by making it difficult for attackers to understand WHERE in code to insert XSS code and other attacks.
In addition, Stored XSS involves server-side input manipulation that ultimately impacts the client side. This makes Stored XSS somewhat unique compared to threats like reverse engineering, where application hardening (e.g., obfuscation and anti-tamper) directly protects the client-side code.
In the case of Stored XSS, obfuscation and anti-tamper techniques are less effective because the core issue lies in the server’s failure to validate and sanitize user input before storing it. The attack occurs when the server inadvertently stores and serves the malicious code, which then runs in the user’s browser. Obfuscation primarily helps secure the code running on the client side from being reverse-engineered or manipulated, but it doesn’t address the injection of malicious code from external sources (like user inputs or database queries).
However, application hardening can still play a complementary role by reducing the potential damage from successful XSS attacks. For example:
Obfuscation makes it harder for attackers to understand and manipulate the client-side code after the XSS script executes. Anti-tamper mechanisms can detect unauthorized changes in the application’s behavior after a successful XSS attack.
Cross-Site Request Forgery (CSRF)
Understanding CSRF Attacks (explain that XSS is required to initiate CSRF)
Cross-Site Request Forgery (CSRF) is an attack that tricks users into performing unintended actions on a web application they have already authenticated. In a typical CSRF attack, the attacker lures a user into clicking a malicious link or visiting a compromised site that sends a forged request to a legitimate web application. Since the user is already authenticated, the application processes the request as if it were legitimate, potentially resulting in actions like changing account settings or initiating transactions without the user’s consent. CSRF is particularly dangerous because the attack leverages the victim’s authentication credentials, making it difficult for the server to distinguish between legitimate and malicious requests. XSS often initiates a CSRF attack, allowing the attacker to inject the malicious script that generates the forged requests. Defending against CSRF requires strict validation of user actions and the use of tokens to verify the legitimacy of each request and/or application hardening, which makes it more difficult for the threat actor to determine where to inject XSS code.
Real-World Examples of CSRF Incidents
CSRF attacks have been behind several high-profile security breaches. Notable examples include:
- YouTube (2008): A CSRF vulnerability allowed attackers to manipulate user settings, such as changing profile details, with a single malicious link.
- Twitter (2010): In a CSRF attack, users were tricked into following others without consent through forged requests.
- Netflix (2006): An attacker could trick users into sending unauthorized requests to their accounts, such as adding or removing DVDs from their rental list.
These incidents illustrate the significant risk CSRF poses to web applications, particularly those that rely heavily on authenticated user sessions. As the line between trusted and untrusted requests can blur, comprehensive mitigation strategies are necessary to defend against these attacks.
Prevention and Mitigation Strategies for CSRF
Anti-CSRF Tokens
One of the most effective methods for preventing Cross-Site Request Forgery (CSRF) attacks is using anti-CSRF tokens. These tokens are unique, randomly generated values associated with each session or request, and they help ensure that a request is legitimate. When a user initiates an action that requires authentication, the server generates a token and embeds it within the request. Upon receiving the request, the server checks whether the token matches the one stored for the user’s session. The server rejects the request if the token is missing, invalid, or incorrect, preventing a potential CSRF attack. Since attackers cannot predict or replicate the correct token, they cannot forge legitimate requests. Anti-CSRF tokens should be applied to all sensitive operations, including form submissions, account modifications, and financial transactions, providing a robust layer of defense against CSRF exploits.
SameSite Cookie Attribute
The SameSite Cookie Attribute is a powerful mechanism for mitigating Cross-Site Request Forgery (CSRF) attacks by controlling how cookies are sent during cross-site requests. By setting the `SameSite` attribute on cookies, you can instruct browsers to only send cookies with requests originating from the same site, thereby preventing attackers from using cookies in cross-site contexts to forge requests. This attribute has three possible settings: Strict, Lax, and None. The Strict setting ensures that cookies are never sent with cross-site requests, offering the strongest protection against CSRF, though it may impact usability in some instances, such as when users need to share links across sites. The Lax setting provides a balance by allowing browsers to send cookies on certain types of safe, top-level navigation requests. In contrast, the None setting enables browsers to send cookies with all requests, including cross-site requests, which developers should use with caution. By leveraging the SameSite attribute effectively, developers can significantly reduce the risk of CSRF attacks while maintaining a functional user experience.
Double Submit Cookies
The Double Submit Cookies technique is another effective strategy for mitigating Cross-Site Request Forgery (CSRF) attacks. In this method, the server issues a CSRF token stored both as a cookie and as a hidden field within forms or headers during requests. When a user submits a form or sends a request, the server checks that the CSRF token matches the token stored in the cookie. Since the attacker cannot modify or control both the cookie and the request content simultaneously, this technique ensures that only valid requests are accepted. Unlike anti-CSRF tokens, Double Submit Cookies don’t require server-side token storage, simplifying the implementation. However, this method is only effective if developers encrypt the communication between the client and server (e.g., over HTTPS) to prevent attackers from intercepting and manipulating the tokens.
Application Hardening (Obfuscation and Anti-Tamper)
Application Hardening, aka techniques like obfuscation and anti-tamper measures, plays a vital role in mitigating the impact of CSRF attacks. By obfuscating JavaScript code, you make it more difficult for attackers to understand the application’s logic or identify potential vulnerabilities they can exploit to inject malicious requests. Anti-tamper techniques can further ensure that unauthorized changes or manipulations to the application’s client-side code are detected and blocked. While these measures don’t directly prevent CSRF, they add an additional layer of security by reducing the likelihood that an attacker can exploit weaknesses in your application’s client-side behavior, especially when used in conjunction with CSRF-specific defenses like tokens and the SameSite cookie attribute.
Clickjacking
What is Clickjacking?
Clickjacking is a deceptive attack where attackers trick users into clicking on something different from what they perceive by overlaying malicious content on top of legitimate elements. They typically embed a transparent or hidden frame within a web page. As a result, users unknowingly interact with attacker-controlled elements—such as buttons or links—when they think they are clicking on something safe, like a video play button or a form submission.
Clickjacking can lead to unintended actions, such as changing security settings, authorizing financial transactions, or granting unauthorized access to sensitive data. The danger of clickjacking lies in its subtlety; users often remain unaware that attackers have manipulated them until the damage is done. As a result, this attack is commonly used in conjunction with other exploits to escalate its impact.
Various Techniques of Clickjacking
Attackers can execute clickjacking through several deceptive techniques, each designed to manipulate user interactions without their knowledge. Common techniques include:
iframe Overlaying | UI Redressing | Cursorjacking | Scrolljacking |
---|---|---|---|
Attackers embed a hidden iframe over a legitimate button or link, causing users to interact unknowingly with the hidden content. | Attackers manipulate the user interface by altering the appearance of a web page, such as making a dangerous button appear harmless. | Attackers misalign the user’s cursor with the actual clickable element, so users believe they are clicking in one spot but are actually interacting with another. | Attackers hijack the scrolling behavior of a page, causing users to unknowingly trigger unintended actions. These subtle clickjacking techniques appear seamless, compromising security without user awareness. |
Prevention and Mitigation Strategies for Clickjacking
X-Frame-Options Header
One of the most effective ways to defend against clickjacking attacks is by implementing the X-Frame-Options header. This HTTP response header instructs the browser whether a page is allowed to be embedded within an iframe on another site. Setting the X-Frame-Options header to `DENY` prevents your web page from being displayed in any iframes, effectively blocking iframe-based clickjacking attacks. Alternatively, you can set the header to `SAMEORIGIN,` which allows the page to be embedded only on the same domain, offering some flexibility while providing protection. Another option is `ALLOW-FROM,` which restricts embedding to specific trusted URLs. This simple yet powerful header is a crucial tool in preventing attackers from embedding your content in a way that deceives users and hijacks their interactions.
Frame-Busting Scripts
Frame-busting scripts are commonly used to prevent a webpage from being loaded inside an iframe, protecting against clickjacking attacks. These scripts detect when a page is being displayed within an iframe and “bust” it out by redirecting it to the top-level window, ensuring it is fully visible to the user. A typical frame-busting script might check if the current page is the top-level window and, if not, force the page to escape the iframe. For example:
`if (window.top !== window.self) window.top.location = window.self.location;`
This is a simple JavaScript snippet that performs this action. While frame-busting scripts offer an additional layer of defense, they can sometimes be bypassed or interfere with legitimate uses of iframes, such as embedding on trusted sites. Therefore, they are best used in combination with other protective measures like the X-Frame-Options header.
Content Security Policy (CSP) Frame Ancestors
The Content Security Policy (CSP) Frame Ancestors directive is another effective way to prevent clickjacking attacks. This CSP directive specifies which origins are allowed to embed your content in an iframe, giving you fine-grained control over where your web pages can be displayed. By setting the `frame-ancestors` directive, you can restrict iframe embedding to specific, trusted domains or block embedding entirely. For example, using:
`Content-Security-Policy: frame-ancestors 'self';`
Ensures that your content can only be embedded on pages from the same domain while:
`Content-Security-Policy: frame-ancestors 'none';`
Blocks all iframe embedding, much like the X-Frame-Options header. The advantage of using the `frame-ancestors` directive over X-Frame-Options is that it’s more flexible and part of the broader CSP framework, which can be extended to cover other security needs. This approach provides robust protection against clickjacking, particularly in modern browsers that fully support CSP.
Application Hardening (Obfuscation and Anti-Tamper)
Application Hardening techniques like obfuscation and anti-tamper measures add a layer of protection by making it harder for attackers to understand or manipulate the client-side code that may be used in a clickjacking exploit. Obfuscation scrambles the code, making it difficult for attackers to reverse-engineer or inject malicious scripts that manipulate iframes or user interactions. Meanwhile, anti-tamper measures help detect and prevent unauthorized modifications to the application’s code, potentially alerting you if an attacker tries to compromise the client-side behavior. These techniques strengthen the overall security of your web application by making it more resistant to manipulation and exploitation.
Man-in-the-Middle (MitM) Attacks
Overview of MitM Attacks
Man-in-the-Middle (MitM) attacks occur when an attacker intercepts and potentially alters communication between two parties, such as between a user and a web application, without either party being aware. These attacks often exploit unsecured or weakly encrypted communication channels, enabling the attacker to eavesdrop on sensitive data, modify messages, or impersonate one of the communicating parties. A common example is an attacker intercepting data exchanged over an unsecured Wi-Fi network. MitM attacks can have severe consequences, such as credential theft, data manipulation, or financial fraud. By positioning themselves between the user and the application, the attacker can capture everything from login credentials to session cookies and, in some cases, inject malicious data into the communication stream. Defending against MitM attacks requires strong encryption, proper authentication, and vigilance in securing network communication.
Common Methods Used in MitM Attacks
H4 HTTP Downgrade Attack
An HTTP Downgrade Attack is a type of Man-in-the-Middle (MitM) attack where an attacker forces a user’s browser to revert from a secure HTTPS connection to an unsecured HTTP connection. By downgrading the connection, the attacker can intercept and manipulate the data transmitted between the user and the web server, as HTTP does not provide the encryption protections that HTTPS does. This attack typically occurs when a server supports HTTP and HTTPS protocols, and the attacker manipulates the communication to downgrade the connection to HTTP. Once the connection is downgraded, the attacker can eavesdrop on sensitive information, inject malicious content, or alter the exchanged data. Strict Transport Security (HSTS) can help mitigate this type of attack by ensuring browsers only connect to the server using HTTPS, even when users try to access the site via HTTP.
SSL Stripping
SSL Stripping is a type of Man-in-the-Middle (MitM) attack where the attacker downgrades a secure HTTPS connection to an insecure HTTP connection without the user’s knowledge. In this attack, the user initiates a connection to a website using HTTPS, but the attacker intercepts the request and forces it to be served over HTTP. As a result, the data transmitted between the user and the web server is no longer encrypted, making it vulnerable to eavesdropping and manipulation. The attacker can intercept sensitive information such as login credentials, session cookies, or personal data. This type of attack is particularly dangerous because the user may believe they are on a secure connection, as the website appears to function normally. HSTS (HTTP Strict Transport Security) is an effective defense against SSL Stripping, ensuring that a browser only connects to a website using HTTPS and disallows any fallback to HTTP, thus mitigating this attack.
Session Hijacking
Session Hijacking is a Man-in-the-Middle (MitM) attack where an attacker gains unauthorized access to a user’s active session with a web application. This attack occurs when an attacker intercepts or steals the session token—a unique identifier stored in a cookie or URL that keeps a user logged in after authentication. Once the attacker obtains this token, they can impersonate the user, gain access to their account, and perform actions on their behalf, such as changing account settings, viewing sensitive information, or conducting unauthorized transactions. Session hijacking is particularly dangerous because it allows attackers to bypass authentication without the user’s credentials. Techniques such as strong encryption of session cookies, regenerating session IDs after login, and using Secure and HttpOnly cookie attributes help protect against session hijacking by making it harder for attackers to steal or misuse session tokens.
Real-World Examples of MiTM Attacks
Man-in-the-Middle (MitM) attacks have caused significant breaches across various industries. Notable examples include:
- Superfish Incident (2015): Lenovo laptops were pre-installed with adware that acted as a proxy, intercepting and altering HTTPS traffic. This allowed attackers to inject ads into websites and exposed users’ secure connections to tampering, opening the door for more severe MitM attacks.
- NSA’s Quantum Insert Technique: Revealed in the Snowden leaks, the NSA used this method to intercept traffic between users and popular websites, injecting malicious content to compromise targeted systems.
- Wi-Fi MitM Attacks: More prevalent than ever, attackers exploit vulnerabilities in public Wi-Fi networks to intercept unencrypted communications, stealing login credentials and other sensitive data from unsuspecting users.
These incidents highlight the importance of encrypting communication and implementing strong authentication mechanisms to prevent unauthorized access.
Prevention and Mitigation Strategies for MitM Attacks
HTTPS Everywhere
One of the most effective ways to protect against Man-in-the-Middle (MitM) attacks is by enforcing HTTPS Everywhere—ensuring that all communication between users and your application is encrypted with HTTPS. HTTPS uses TLS (Transport Layer Security) to secure data in transit, preventing attackers from intercepting or manipulating the information exchanged between the client and server. By implementing HTTPS across your entire site, including all subdomains, and redirecting all HTTP traffic to HTTPS, you protect users from attackers who might attempt to downgrade connections or intercept unencrypted data. HSTS (HTTP Strict Transport Security) helps enforce this policy by instructing browsers to only connect to the site using HTTPS, even if the user accidentally tries to connect via HTTP. In today’s environment, using HTTPS Everywhere is a foundational security measure to defend against MitM attacks.
Strong Encryption Protocols
Strong encryption protocols are critical to defending against Man-in-the-Middle (MitM) attacks, as they ensure that any data transmitted between the client and server is unreadable to attackers who intercept it. TLS (Transport Layer Security) is the industry standard for encrypting web traffic and should be configured to use modern, strong encryption algorithms such as AES (Advanced Encryption Standard) with 256-bit keys. Deprecated protocols like SSL (Secure Sockets Layer) and older versions of TLS should be avoided, as they have known vulnerabilities that can be exploited in MitM attacks. Additionally, forward secrecy should be enabled, which ensures that even if a key is compromised, past communications remain secure. By implementing robust encryption protocols, organizations can protect sensitive data, such as login credentials and payment information, from being stolen or tampered with during transmission.
Certificate Pinning
Certificate pinning is an advanced security measure that prevents Man-in-the-Middle (MitM) attacks by ensuring that a client (such as a web browser or mobile app) only accepts a specific, trusted certificate when communicating with a server. In a typical HTTPS connection, the browser verifies the server’s certificate with trusted certificate authorities (CAs). However, attackers can exploit vulnerabilities in the CA system or use fraudulent certificates to intercept traffic. With certificate pinning, you “pin” a specific certificate or public key to your app or browser, ensuring that only the legitimate certificate is accepted, even if an attacker presents a different, valid certificate from a trusted CA. This reduces the risk of attackers using fake or compromised certificates to intercept encrypted communications. While certificate pinning strengthens security, it needs careful implementation and maintenance, especially during certificate updates, to avoid unintentionally blocking legitimate connections.
Application Hardening (Obfuscation and Anti-Tamper)
Application Hardening techniques, such as obfuscation and anti-tamper, can play a role in reducing the risk of Man-in-the-Middle (MitM) attacks, especially in client-side applications. Obfuscating the code makes it more difficult for attackers to reverse-engineer the application and identify potential weaknesses they can exploit in a MitM attack. Anti-tamper mechanisms can detect and prevent unauthorized changes to the application, making it harder for attackers to inject malicious code or modify the app’s behavior during communication. Additionally, by implementing runtime checks and app monitoring, application hardening can detect suspicious activity or manipulation attempts that may indicate a MitM attack is in progress. While these techniques do not directly encrypt or secure the communication channel, they make it harder for attackers to gain insights into or manipulate the application, adding an important layer of defense.
White Box Cryptography
White-box cryptography enhances security in Man-in-the-Middle (MitM) attack prevention by protecting cryptographic keys and operations within client-side applications. By embedding and obfuscating encryption keys within the cryptographic process, white-box cryptography ensures that attackers cannot extract or manipulate sensitive data even if they have full access to the client environment. It strengthens the integrity of communications, protects key exchange mechanisms, and prevents attackers from tampering with or injecting malicious code into the communication stream. While it doesn’t replace traditional encryption protocols, white-box cryptography adds an additional layer of security, making it harder for attackers to compromise client-side code in a MitM scenario.
Malicious Browser Extensions and Plugins
Risks Posed by Malicious Browser Extensions
Malicious browser extensions pose a significant threat to client-side security, as they often have elevated permissions and access to sensitive data within the browser. Once installed, these extensions can intercept and manipulate web traffic, steal login credentials, inject malicious scripts into web pages, or track users’ online behavior without their consent. Because extensions run in the user’s browser, they can access everything from cookies and session data to personal information entered into forms. Attackers can also use malicious plugins to bypass security measures implemented by websites, such as encryption, allowing them to intercept sensitive communications or alter webpage content. Even trusted extensions can be hijacked or compromised by attackers, turning them into vectors for distributing malware or launching Man-in-the-Middle (MitM) attacks. Given the elevated privileges extensions can possess, they represent a critical risk to the security of users and the applications they interact with.
Notable Incidents Involving Malicious Extensions
Several high-profile incidents have highlighted the dangers of malicious browser extensions:
Shitcoin Wallet Chrome Extension (2020) | Removal of 500+ Chrome Extensions (2019) | MEGA’s Chrome Extension (2018) | WebEx Chrome Extension Vulnerability (2017) |
---|---|---|---|
The extension was found to be stealing cryptocurrency wallet private keys and passwords by injecting malicious JavaScript into web pages. | The extensions were removed from the Chrome Web Store after they were found to be part of a massive malware distribution network, injecting ads and stealing user data. | Although a trusted extension, it was compromised when attackers hijacked it to steal credentials and private keys. | The vulnerability allowed attackers to remotely execute arbitrary code on users’ devices by exploiting a flaw in the extension’s handling of web requests. |
These incidents demonstrate the far-reaching impact of malicious or compromised browser extensions, showing how they can be exploited to steal data, compromise accounts, and launch widespread user attacks.
Prevention and Mitigation Strategies
User Awareness and Education
One of the most important strategies for preventing the installation of malicious browser extensions is raising user awareness and providing education about the risks involved. Many users are unaware that browser extensions can have extensive access to their personal data and interactions with web applications. Educating users on the importance of installing extensions only from trusted, verified sources can significantly reduce the risk of falling victim to malicious plugins. Additionally, users should be informed about reviewing the permissions requested by extensions. If an extension asks for excessive access, such as the ability to read and modify all data on websites visited, it may be a red flag. Regular training and awareness campaigns can also encourage users to report suspicious behavior and be cautious about clicking links or downloading software from unknown sources. Ultimately, organizations can strengthen their overall security posture by empowering users to recognize the warning signs of potentially harmful extensions.
Trusted Extension Sources
Installing browser extensions from trusted and verified sources is one of the most effective ways to prevent the installation of malicious or compromised extensions. Extensions should always be downloaded from official extension stores, such as the Chrome Web Store or Mozilla Add-ons, where submissions are vetted for security. However, even extensions from trusted stores are not immune to compromise, as attackers sometimes slip malicious updates through or hijack previously legitimate extensions. To mitigate this, organizations can create a whitelist of approved extensions that have been thoroughly vetted for security and ensure that users only install extensions from this trusted list. Additionally, keeping track of trusted developers and publishers helps users recognize extensions from reliable sources. By emphasizing the use of trusted extension sources, users and organizations can significantly reduce the risk of introducing malicious code into their browsers.
Regular Security Audits
Conducting regular security audits on installed browser extensions is crucial for detecting and removing malicious or compromised extensions before they can cause harm. Security audits should involve reviewing the permissions each extension has access to, ensuring they align with the intended functionality. Organizations can use automated tools to scan for extensions with known vulnerabilities, excessive permissions, or suspicious behavior. Auditing should also include monitoring for unusual traffic or data exfiltration that might indicate an extension is acting maliciously. Regularly reviewing and removing unnecessary or outdated extensions reduces the attack surface. In environments where sensitive data is handled, such as finance or healthcare, these audits can be automated as part of broader security assessments to ensure malicious plugins do not become a backdoor into critical systems.
Application Hardening (Obfuscation and Anti-Tamper)
Application Hardening can help mitigate the risks posed by malicious browser extensions by making it more difficult for attackers to manipulate or reverse-engineer the client-side code of web applications. Techniques like code obfuscation hide the structure and logic of the application, making it harder for extensions to inject malicious code or interfere with sensitive operations. Anti-tamper mechanisms can detect unauthorized changes in the application, alerting developers to potential compromises caused by malicious extensions. Additionally, integrating runtime application self-protection (RASP) can further enhance security by continuously monitoring and responding to suspicious activity, ensuring that the application remains resilient even if a malicious extension attempts to alter its behavior. Application hardening adds an extra layer of defense, complementing other strategies to prevent extension-based threats.
Local Storage and Session Storage Vulnerabilities
Understanding Local and Session Storage
Local and session storage are two client-side web storage mechanisms that allow browsers to store data directly on the user’s device. Both are part of the Web Storage API, which enables web applications to store key-value pairs in a more persistent manner compared to cookies, without affecting server-side interactions. Local storage stores data with no expiration time, meaning the information remains accessible even after the browser is closed and reopened. In contrast, session storage only persists for the duration of the page session, meaning the data is cleared as soon as the user closes the browser tab. Local and session storage are useful for enhancing user experience by storing data like preferences or session states.
Security Risks Associated with Client-Side Storage
While both local and session storage are useful for enhancing user experience by storing data like preferences or session states, they also introduce security risks. Since they are stored in the browser, they are fully accessible to any JavaScript running on the page, including potentially malicious scripts, making them prime targets for cross-site scripting (XSS) attacks or other client-side threats.
Prevention and Mitigation Strategies
Encryption of Sensitive Data
Encrypting sensitive data stored in local or session storage is a critical step in protecting it from unauthorized access, especially in the context of client-side vulnerabilities like cross-site scripting (XSS) or other injection attacks. By encrypting data before storing it in the browser, even if an attacker manages to access the storage, the data will remain unintelligible without the correct decryption key. White-box cryptography can further enhance this protection by embedding cryptographic keys directly into the application in a way that makes them difficult to extract or reverse-engineer, even if the attacker has full access to the client-side environment. This ensures that sensitive data, such as authentication tokens or personal information, is secured against client-side attacks. Implementing encryption in combination with secure key management practices helps mitigate the risks associated with storing sensitive data in local or session storage, ensuring the data remains protected even in a compromised browser environment.
Expiration of Sensitive Data
It’s important to enforce strict expiration policies for data stored in local or session storage to reduce the risk of sensitive information being exposed in client-side storage. Sensitive data should only be stored for as long as it is absolutely necessary. For example, session data should expire when the user closes the browser, and local storage data should be configured to expire after a set period of inactivity. This minimizes the window of opportunity for attackers to access sensitive information. Implementing expiration mechanisms helps ensure that stale or unnecessary data isn’t left accessible to potential threats, reducing the risk of data leakage or misuse in case of a client-side attack.
Regular Auditing and Access Controls
To ensure the security of data stored in local and session storage, organizations should implement regular audits and strong access controls. Regular audits help identify and remove any sensitive data that should no longer be stored, ensuring that only necessary information is retained. These audits should also look for potential security weaknesses or unusual patterns of access that could indicate malicious activity. Access controls are equally important, ensuring that only authorized scripts and users can interact with sensitive data. This can be achieved by restricting JavaScript execution to trusted domains and using content security policies (CSPs) to prevent unauthorized access. By combining regular audits with robust access controls, organizations can significantly reduce the risks associated with storing sensitive data in the browser, ensuring that only approved processes have access to critical information.
Application Hardening (Obfuscation and Anti-Tamper)
Application Hardening techniques, such as code obfuscation and anti-tamper measures, play a crucial role in protecting data stored in local and session storage by making it more difficult for attackers to exploit client-side vulnerabilities. Obfuscation helps obscure the application’s logic, making it harder for attackers to reverse-engineer code and understand how sensitive data is handled or accessed. Anti-tamper mechanisms can detect and block unauthorized attempts to modify the application’s code, preventing attackers from altering the way the app stores or retrieves sensitive data. Additionally, runtime protection ensures that if malicious actors manage to compromise the storage, the application can respond by detecting unusual behaviors and taking action to prevent data theft or further exploitation. By hardening the application, developers can significantly raise the bar for attackers, making it much harder to exploit weaknesses in client-side storage.
Third-Party Scripts and Dependencies
Risks of Using Third-Party Scripts
Using third-party scripts introduces significant security risks to web applications, as these external dependencies can become attack vectors for malicious actors. Since third-party scripts often have extensive access to a website’s functionality and user data, they can be exploited if compromised. For example, attackers can inject malicious code into scripts hosted on third-party servers, turning a trusted script into a tool for data theft, malware distribution, or unauthorized actions on the client side. Moreover, developers often have limited visibility into the internal workings of third-party scripts, making it harder to detect vulnerabilities or malicious behavior. This lack of control is compounded by the risk of supply chain attacks, where attackers compromise a third-party provider, affecting thousands of websites that rely on the provider’s scripts. These risks make it critical for organizations to carefully evaluate and monitor all third-party dependencies to mitigate potential security threats.
Real-World Examples of Compromised Third-Party Scripts
In recent years, compromised third-party scripts have been behind some of the most damaging cyberattacks. Notable examples include:
- British Airways Magecart Attack (2018): Attackers injected malicious code into the payment page, stealing the payment information of over 380,000 customers. The attackers exploited a vulnerability in third-party scripts used by the airline, leading to the breach.
- Ticketmaster Magecart Attack (2018): Malicious JavaScript was injected through third-party vendors, compromising payment data from thousands of customers.
- Amazon S3 Buckets Compromise: Attackers exploited misconfigured storage to inject malicious scripts into thousands of websites, impacting 17,000 domains.
These incidents highlight the serious risks associated with using third-party scripts, as even a single vulnerability can lead to widespread data theft and security breaches.
Prevention and Mitigation Strategies
Subresource Integrity (SRI)
Subresource Integrity (SRI) is a security feature that helps ensure the integrity of external scripts and resources loaded by a website. By specifying a cryptographic hash in the HTML tag that references a third-party script, SRI enables browsers to verify that the script hasn’t been altered during transmission. If the script’s content has been modified, the browser will reject it, preventing the execution of potentially malicious code. However, SRI has important limitations. It only protects against changes in the script during transmission—such as a man-in-the-middle attack or CDN compromise—but does not safeguard against vulnerabilities in the original source itself. If the third-party script is compromised at its source or a developer incorrectly updates the hash, SRI won’t prevent the attack. Therefore, while SRI adds a valuable layer of security, it must be combined with regular audits, application hardening, and other client-side protections to ensure comprehensive protection against third-party script risks.
Deferred Loading of Scripts
Deferred loading of scripts is a technique that can improve both performance and security by delaying the execution of non-critical third-party scripts until after the page’s main content has loaded. This ensures that essential content is prioritized, while potentially risky third-party scripts are only loaded when absolutely necessary. From a security standpoint, deferring the loading of third-party scripts reduces the window of opportunity for attackers to exploit vulnerabilities during the initial page load. This method also allows developers to more carefully assess and monitor the behavior of third-party scripts before they run, especially in cases where user interaction is required. However, deferred loading should be used with other security strategies, such as Subresource Integrity (SRI), as it doesn’t prevent malicious scripts from being loaded if they are already compromised. By deferring script execution, you give users faster access to critical content while reducing the immediate risks posed by third-party scripts.
Application Hardening (Obfuscation and Anti-Tamper)
Application Hardening techniques, such as obfuscation and anti-tamper, are crucial for protecting web applications from the risks associated with compromised third-party scripts. By making the underlying client-side code more difficult to understand, obfuscation adds a layer of complexity for attackers attempting to exploit or manipulate your application’s interactions with third-party resources. Anti-tamper measures further enhance security by detecting unauthorized modifications to the application’s code at runtime, ensuring that any attempts to inject or alter malicious scripts are blocked.
Phishing and Social Engineering Attacks
Overview of Phishing Techniques
Phishing is a widely used cyberattack method that tricks users into divulging sensitive information, such as login credentials or financial details, by pretending to be a legitimate entity. Phishing links often direct victims to fake websites designed to capture personal information. Common phishing techniques include:
Email Phishing | Spear Phishing | Pharming |
---|---|---|
Attackers send fraudulent emails or text messages, mimicking trusted organizations but containing malicious links. | Targets specific individuals with more personalized messages, making the scam seem more authentic. | Redirects users to malicious websites without their knowledge by manipulating browser settings or DNS queries. |
In the context of client-side security threats, phishing is especially relevant because it exploits the user’s interaction with the browser and client-side applications. Attackers may inject malicious scripts into client-side code or compromise trusted web resources to deliver phishing pages. Even well-protected web applications can fall victim to phishing attacks if users are lured into providing their credentials on spoofed sites. Thus, defending against phishing is critical in any comprehensive client-side security strategy.
Common Social Engineering Tactics
Social engineering is a manipulation technique attackers use to exploit human psychology and trick victims into divulging confidential information or performing unauthorized actions. Beyond the aforementioned phishing tactics, some common tactics include:
Pretexting | Baiting | Quid Pro Quo |
---|---|---|
The attacker invents a believable scenario to gain trust, such as impersonating a technical support representative or a company executive. | The attacker uses the promise of something enticing, like free software or a prize, to lure victims into clicking on a malicious link or downloading malware. | The attacker offers something in exchange for information, such as posing as an IT professional offering free assistance. |
All these tactics prey on trust, curiosity, and urgency, making them effective at bypassing technological defenses and directly compromising users on the client side.
Prevention and Mitigation Strategies
User Education and Awareness Training
Educating users about phishing and social engineering tactics is one of the most effective ways to mitigate client-side security threats. Comprehensive user education should focus on helping individuals recognize suspicious emails, text messages, and pop-ups that may be phishing attempts. Training programs should emphasize the importance of verifying the authenticity of links, avoiding clicking on unknown attachments, and reporting suspicious communications. Additionally, users should be encouraged to look for signs of spoofed websites, such as incorrect URLs or unsecured connections, before entering sensitive information. Awareness training can also help users spot social engineering techniques, such as urgent requests for personal information, and reduce their likelihood of falling victim to such attacks. Regular phishing simulations and security reminders reinforce these lessons, ensuring users remain vigilant and prepared to respond effectively to evolving threats.
Multi-Factor Authentication (MFA)
Multi-Factor Authentication (MFA) is a critical security measure that helps protect users from phishing and social engineering attacks by requiring multiple forms of verification before granting access to sensitive accounts or systems. With MFA, even if an attacker manages to steal a user’s credentials through phishing, they cannot gain access without passing an additional verification step, such as a one-time password (OTP), biometric authentication, or a push notification sent to a trusted device. This added layer of security significantly reduces the risk of unauthorized access, as attackers would need to compromise not only the password but also the secondary authentication factor. Implementing MFA across all critical systems and user accounts strengthens the overall security posture and limits the damage potential from phishing or compromised credentials. Encouraging users to enable MFA for both personal and work accounts helps protect sensitive information, even if one form of authentication is compromised.
Secure Email Gateways
A Secure Email Gateway (SEG) is an essential defense against phishing attacks and other email-based threats. It filters and blocks malicious emails before they reach users’ inboxes. SEGs analyze incoming and outgoing emails for suspicious content, attachments, and URLs, flagging or quarantining emails that contain known malware, phishing links, or other harmful elements. Advanced SEGs also use machine learning and behavioral analysis to detect more sophisticated attacks, such as spear phishing or business email compromise (BEC). In addition to preventing phishing attacks, SEGs actively enforce policies and implement data loss prevention (DLP) features to stop sensitive information from being inadvertently sent via email. By implementing a secure email gateway, organizations can drastically reduce the likelihood of phishing attempts reaching their users and minimize the risk of successful client-side security breaches initiated through email.
Progressive Web App (PWA) Security Threats
Unique Threats to PWAs
Progressive Web Applications (PWAs) combine the best features of web and mobile applications, providing users with a seamless experience across platforms. However, this hybrid nature introduces unique security threats. Since PWAs can be installed directly from the browser, bypassing traditional app stores, they may not undergo the rigorous security vetting that native mobile apps face. Additionally, PWAs rely heavily on service workers—scripts that run in the background to handle caching, push notifications, and offline functionality. If a service worker is compromised, it can manipulate the PWA’s behavior, intercept sensitive data, or serve malicious content. PWAs also interact with client-side storage, such as IndexedDB and local storage, which can be vulnerable to cross-site scripting (XSS) attacks. Finally, PWAs use APIs that allow access to device features like the camera or location, making them potential targets for privacy invasions or malicious actions if exploited. These unique challenges make it essential to apply robust security practices, including secure API implementation and rigorous validation of service workers.
Real-World Attacks on PW Applications
In recent years, attacks targeting Progressive Web Apps (PWAs) have seen a rise, leveraging their unique characteristics to deceive users. Notable examples include:
- Banking App Impersonation (2023): Cybercriminals launched phishing campaigns using PWAs disguised as legitimate banking apps. These attacks, observed in countries like Poland and Hungary, involved PWAs mimicking real banking interfaces on Android and iOS devices. By bypassing app store vetting processes, attackers could distribute these fake apps via malicious ads and phishing links, leading users to unknowingly install compromised PWAs that stole their banking credentials.
- Phishing Toolkit with Fake Login Forms: Attackers leveraged PWAs to display convincing login forms, even embedding fake address bars to make them appear legitimate.
These attacks demonstrate how PWAs can be exploited with their access to device features and app-like behavior to carry out highly effective phishing and credential-stealing campaigns.
Best Practices for Securing PWA Applications
Yes, application hardening can play an important role in the safe implementation of Web APIs for Progressive Web Applications (PWAs). By applying techniques like code obfuscation, anti-tamper mechanisms, and runtime application self-protection (RASP), developers can make it more difficult for attackers to reverse-engineer or exploit API calls within the PWA.
How Application Hardening Helps:
- Code Obfuscation: Obfuscating the PWA’s code makes it much harder for attackers to understand how API calls are structured or find weak points they can exploit. This ensures critical API interactions, like those handling sensitive data (e.g., geolocation, payment, or authentication APIs), are more resistant to analysis and tampering.
- Anti-Tamper Mechanisms: These mechanisms help detect and prevent unauthorized changes to the code or API implementations. If an attacker attempts to modify how the PWA communicates with its APIs, anti-tamper solutions can flag the changes, block execution, or trigger alerts.
- Runtime Protection (RASP): RASP monitors the application while it’s running, providing real-time protection against exploitation attempts, including attempts to misuse APIs. If an API is used unexpectedly or maliciously, runtime protection can detect the anomaly and halt the process before it leads to a security breach.
By combining secure coding practices with application hardening, developers can significantly reduce the attack surface of Web APIs in PWAs, making them less vulnerable to reverse engineering, tampering, or unauthorized access.
As web applications become more sophisticated and widely used, client-side security threats are increasingly prevalent and dangerous. Attackers exploit vulnerabilities in the client-side environment through techniques like Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), Man-in-the-Middle (MitM) attacks, and malicious browser extensions. As seen with the rise of Progressive Web Applications (PWAs), attackers continue to develop innovative methods, such as phishing campaigns that mimic legitimate apps, targeting unsuspecting users. These threats underscore the importance of securing client-side code and interactions, especially since they directly impact users and sensitive data.
A comprehensive security strategy is essential to mitigate these risks. This includes adopting techniques like input validation, output encoding, and Content Security Policies (CSP) to protect against XSS, using Anti-CSRF tokens and SameSite cookie attributes for CSRF defense, and employing robust encryption protocols, certificate pinning, and application hardening to protect against MitM attacks. Additionally, safeguarding local and session storage with encryption and regular audits, as well as securing third-party scripts and dependencies with strategies like Subresource Integrity (SRI), is crucial to maintaining the integrity of your application.
User awareness, especially around phishing and social engineering, remains a cornerstone of client-side security. Training users to recognize suspicious activity, combined with strong technical defenses like Multi-Factor Authentication (MFA) and secure email gateways, can dramatically reduce the likelihood of successful attacks.
Finally, application hardening—through techniques like obfuscation and anti-tamper—provides a critical layer of security across all these areas, making it more difficult for attackers to reverse-engineer or exploit client-side code. Developers should continuously evaluate and update their security practices as the threat landscape evolves to stay ahead of emerging threats.
By combining technical measures, user education, and regular auditing, organizations can significantly reduce their exposure to client-side threats and build more resilient web applications that protect their data and users.
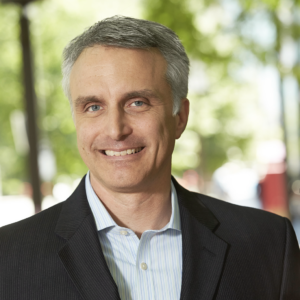
Application Security for Web
Explore
What's New In The World of Digital.ai
Announcing Quick Protect Agent: MASVS-Aligned Protections, Now Easier Than Ever
Easily apply OWASP MASVS-aligned protections to your mobile apps—no coding needed. Quick Protect Agent delivers enterprise-grade security in minutes.
“Think Like a Hacker” Webinar Recap: How AI is Reshaping App Security
Discover how generative AI is reshaping app security—empowering both developers and hackers. Learn key strategies to defend against AI-powered threats.
The Encryption Mandate: A Deep Dive into Securing Data in 2025
Discover how white-box cryptography and advanced encryption help enterprises secure sensitive data, meet compliance, and stay ahead of cybersecurity threats.