Table of Contents
Related Blogs
iOS apps are ripe targets for threat actors. Developers can safeguard their apps against reverse engineering, unauthorized code manipulation, and other emerging threats by implementing robust security practices. This guide will explore essential best practices for iOS app security, covering everything from code obfuscation and data encryption to runtime protection and secure app distribution.
Overview of iOS Security Architecture
The iOS security architecture is designed to provide a layered defense system, protecting apps and user data from unauthorized access and malicious attacks. Core features such as hardware-backed encryption, secure boot chains, and sandboxing create a strong foundation, but securing an app requires more than the platform’s built-in protections. Application hardening is a critical component, employing techniques like:
- Code obfuscation to conceal app logic
- Anti-tamper mechanisms to detect and resist unauthorized modifications
- Runtime Application Self-Protection (RASP) to monitor and defend the app during execution
- Robust threat monitoring tools provide real-time insights into potential attacks, empowering developers to respond swiftly to emerging risks.
By layering these measures on top of iOS’s native security, developers can build resilient apps against common and sophisticated threats.
Importance of App Security in the iOS Ecosystem
In the iOS ecosystem, an ecosystem increasingly interwoven with the everyday personal, private, recreational, and business lives of all of us, security is paramount. Apple’s ecosystem offers robust native security features, including strict App Store review processes and device-level protections–but these measures alone cannot and do not safeguard against sophisticated threats or even many common threats. Apps remain vulnerable to reverse engineering, unauthorized code manipulation, and runtime attacks, particularly as threat actors become more sophisticated. Securing iOS apps with advanced measures like application hardening protects user data, maintains brand reputation, and ensures compliance with privacy regulations. In a competitive market, strong app security isn’t just a technical necessity—it’s a business imperative.
Understanding Threats to iOS Apps
Common Vulnerabilities in iOS Applications
Despite the robust security features of the iOS ecosystem, applications can still exhibit vulnerabilities that expose them to attacks. A lack of obfuscation makes it easier for attackers to reverse-engineer an app’s code, uncovering sensitive logic or keys embedded within it. Similarly, the absence of anti-tamper mechanisms leaves apps vulnerable to unauthorized modifications, such as injecting malicious code or bypassing security checks. Other common vulnerabilities include insecure data storage, weak encryption practices, and insufficient input validation, which can lead to data leaks or exploitation. Failure to implement runtime protections also exposes apps to real-time threats such as dynamic instrumentation and debugging attacks. Addressing these vulnerabilities is essential to protecting both user data and app integrity.
Types of Attacks Targeting iOS Apps
Sophisticated attacks increasingly target iOS apps to exploit weaknesses in-app security.
- Reverse engineering: A common attack where threat actors decompile the app to study its code, uncover sensitive information, or replicate its functionality.
- Code injection and tampering: Allows attackers to modify the app’s behavior, potentially bypassing security controls or embedding malicious functionality.
- Dynamic instrumentation attacks: Often using tools like Frida or Ghidra, enable real-time manipulation of app processes, bypassing authentication or extracting sensitive data.
- Man-in-the-middle (MitM) attacks: Target insecure network communications, intercepting data transmitted between the app and its backend servers.
- Malware and phishing campaigns: Exploit weak app security to steal user credentials or deploy harmful software.
These attack vectors highlight the critical need for comprehensive measures like application hardening, encryption, and runtime monitoring to defend against evolving threats.
Overview of Securing iOS Apps
Secure Coding Practices
Adopting secure coding practices is basic hygiene. Developers should prioritize minimizing attack surfaces by validating all inputs, sanitizing user data, and employing parameterized queries to prevent injection attacks. Sensitive data, such as API keys or cryptographic secrets, should never be hardcoded into the app, as these can be easily extracted through reverse engineering. Leveraging native iOS security APIs, such as Keychain for secure data storage and Secure Enclave for cryptographic operations, adds an additional layer of protection. Regular code reviews, static analysis, and automated testing help identify and remediate vulnerabilities early in the development lifecycle. By writing clean, secure, and defensively structured code, developers can build apps that are inherently more resilient to attacks and reduce the work required of security and test engineers.
Data Protection and Encryption
Data protection and encryption are essential to securing iOS apps and safeguarding sensitive user information. Encryption ensures that data remains unreadable to unauthorized parties, both at rest and in transit. iOS provides built-in frameworks like CommonCrypto and CryptoKit, for implementing strong encryption algorithms like AES-256. Data stored locally on the device should leverage the Keychain or use file protection classes to tie access to the user’s authentication credentials. Implementing secure network protocols such as HTTPS with TLS for data in transit is critical to prevent interception or tampering through man-in-the-middle (MitM) attacks. Incorporating white-box cryptography can add another layer of defense, securing cryptographic keys even if the app is reverse-engineered. By combining robust encryption techniques with proper key management, developers can ensure that sensitive data remains protected against unauthorized access and theft.
Utilizing Apple’s Security Features
Apple provides a comprehensive suite of security features that developers can leverage to enhance the protection of their iOS applications.
Feature | Description |
---|---|
App Transport Security | Enforces secure network connections by requiring apps to use HTTPS, safeguarding data in transit. |
Keychain Services API | Offers a secure way to store sensitive data, such as authentication tokens and passwords. |
Face ID and Touch ID | Secure biometric authentication that adds convenience without compromising security. |
Secure Enclave | A dedicated hardware-based module that ensures cryptographic operations and sensitive data are isolated from the main processor. |
Notarization and App Store Review Processes | Ensures apps are free from known malicious code. |
By integrating these features into their apps, developers can build a robust security foundation aligned with the iOS ecosystem’s best practices.
Regular Security Audits and Testing
Regular security audits and testing are crucial for maintaining the integrity of iOS applications.
- Security audits systematically review the app’s architecture, codebase, and configurations to identify and address potential weaknesses.
- Automated tools like static application security testing (SAST) and dynamic application security testing (DAST) can uncover issues during development and runtime, respectively.
- Penetration testing simulates real-world attacks to evaluate the app’s defenses against advanced threats.
- Integrating continuous security testing into the CI/CD pipeline ensures that updates and new features do not introduce vulnerabilities.
Regularly reviewing dependencies and libraries for known security issues is equally important, as third-party components can be exploited if left unpatched. Developers can proactively address security gaps by committing to ongoing audits, and testing and fortifying their apps against evolving threats.
Application Hardening
Obfuscation
Obfuscation is a critical technique in application hardening that enhances the security of iOS apps by making their code more difficult to understand and reverse-engineer. This process involves transforming readable code into a functionally identical form incomprehensible to threat actors. Techniques such as renaming variables, methods, and classes to non-descriptive terms and inserting misleading or redundant code can effectively obscure the app’s logic. Obfuscation safeguards sensitive information like API keys, algorithms, and proprietary logic, reducing the risk of attackers exploiting or replicating them. While obfuscation alone cannot fully prevent reverse engineering, it significantly raises the difficulty level for attackers, buying valuable time and complementing other security measures such as encryption and anti-tamper techniques.
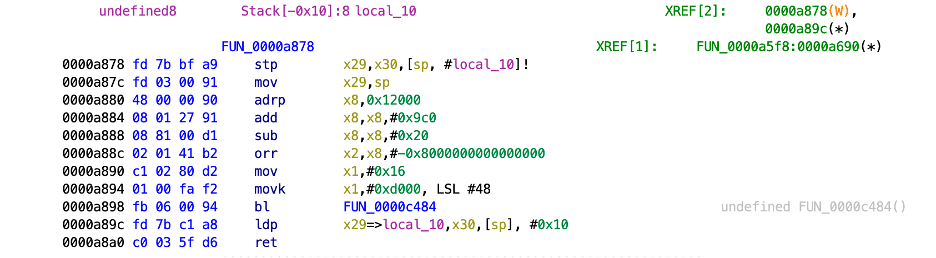
Figure 1: A snippet of Assembly code before obfuscation
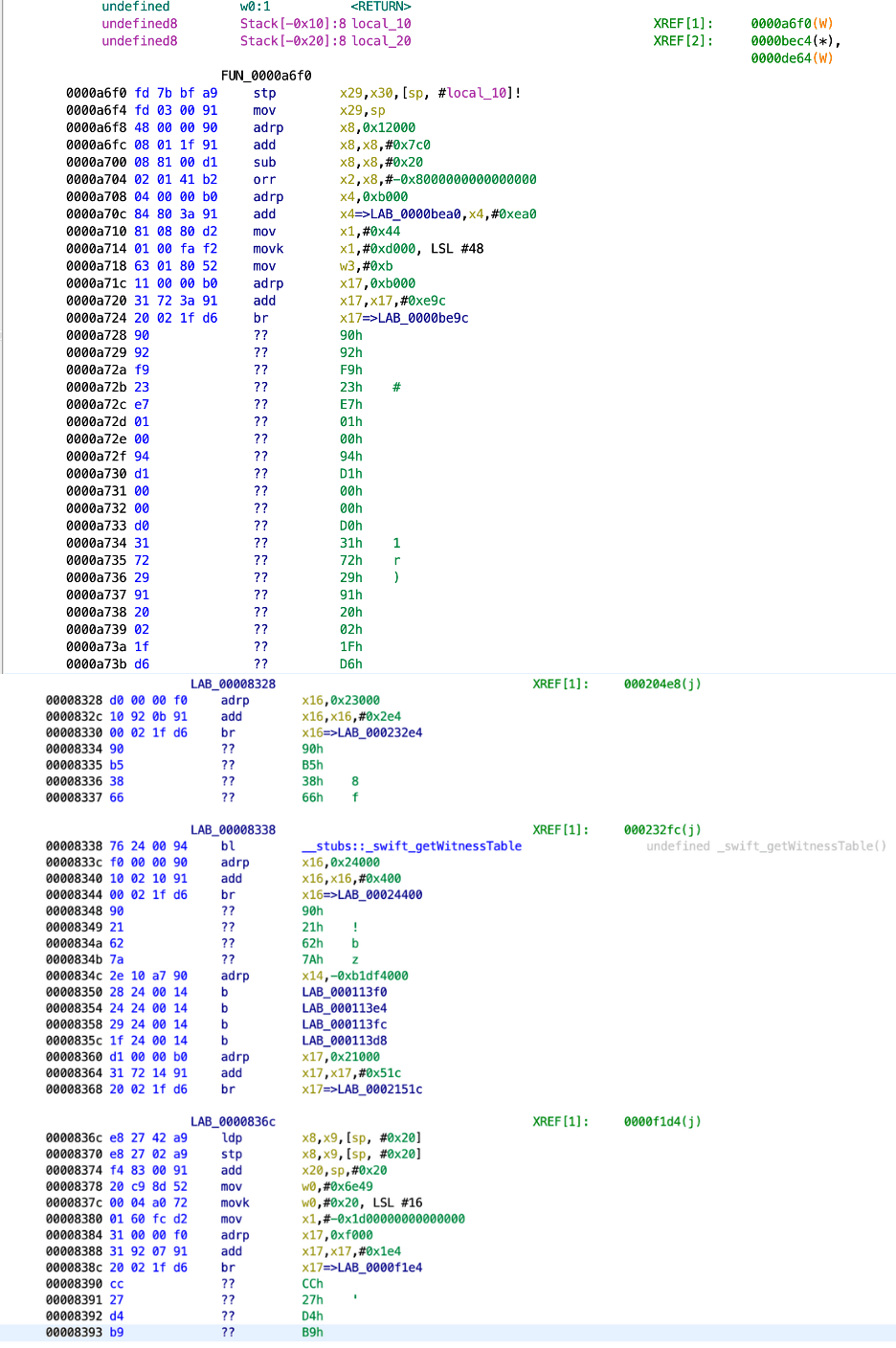
Figure 2: The same snippet of Assembly code after obfuscation
Anti-Tamper
Anti-tamper measures are essential in application hardening to protect iOS apps from unauthorized modifications and malicious interference. These techniques detect and respond to attempts to alter an app’s code, resources, or behavior. Common anti-tamper strategies include:
- Embedding integrity checks to verify the app’s code at runtime, ensuring no unauthorized changes have been made.
- Digital signature validation can confirm the authenticity of the app and its components.
- Anti-tamper mechanisms monitor for signs of debugging or instrumentation tools, triggering alerts or halting app execution when tampering is detected.
By integrating these measures, developers can deter attackers, maintain control over their apps, and protect sensitive data and functionality from being compromised.
Threat Monitoring
Threat monitoring is a proactive approach to securing iOS apps by continuously observing their behavior and environment for signs of malicious activity. This involves embedding tools within the app that can detect unusual patterns, such as attempts to reverse-engineer the code, unauthorized access to sensitive data, or the presence of debugging and dynamic instrumentation tools. Real-time monitoring systems can log suspicious activities, send alerts, and trigger protective responses like restricting app functionality or terminating sessions. Integrating threat monitoring with a centralized Security Information and Event Management (SIEM) system enables developers to gain valuable insights into attack patterns and respond swiftly to emerging threats. Developers can maintain a robust defense by continuously monitoring threats, ensuring the app remains secure despite evolving attack strategies.
RASP
Runtime Application Self-Protection (RASP) is a cutting-edge security measure that enables iOS apps to detect and respond to threats in real time. Unlike traditional perimeter defenses, RASP operates within the app, monitoring its behavior and the execution environment to identify anomalies or malicious activities. RASP can take immediate action when a threat is detected—such as an attempt to inject malicious code, manipulate the app through dynamic instrumentation tools, or exploit a vulnerability. This might include blocking the operation, logging the event, alerting the security team, or shutting down the app to prevent further compromise. By integrating RASP, developers can ensure that their apps remain resilient against sophisticated attacks, providing a dynamic layer of protection that evolves with the threat landscape.
Authentication and Access Control
Implementing Strong Authentication Mechanisms
Strong authentication mechanisms are fundamental to securing iOS applications and ensuring that only authorized users can access sensitive features or data. Implementing multi-factor authentication (MFA), which combines something the user knows (password), something they have (a device or token), and something they are (biometric data), significantly enhances security. iOS provides robust APIs for biometric authentication, such as Face ID and Touch ID, which add a convenient yet highly secure layer of protection. Developers should also enforce secure password policies, such as requiring strong, unique passwords and utilizing hashed storage with algorithms like PBKDF2 or Argon2. Additionally, integrating federated identity providers through protocols like OAuth 2.0 or OpenID Connect can streamline authentication while leveraging the security of established platforms. By prioritizing strong authentication, developers can greatly reduce the risk of unauthorized access and ensure a safer user experience.
Role-Based Access Control and Permissions Management
Role-Based Access Control (RBAC) and effective permissions management are crucial for maintaining security within iOS applications. RBAC assigns specific roles to users based on their responsibilities, ensuring they only have access to the features and data necessary for their role. For example, an admin might have access to configuration settings, while regular users can only view or interact with specific app functions. Developers should implement fine-grained permissions management, where access is granted or denied at the resource level, minimizing exposure of sensitive data. iOS frameworks like NSUserDefaults and Keychain Services can securely store and manage permission settings, while server-side logic ensures consistency across sessions and devices. Regularly reviewing and updating roles and permissions as user needs evolve further strengthens security, ensuring a principle of least privilege is consistently applied.
Network Security for iOS Apps
Ensuring Secure Network Communications
Secure network communications are essential for protecting data exchanged between iOS apps and their backend servers. Using HTTPS with TLS (Transport Layer Security) ensures that data is encrypted during transit, safeguarding it from interception in man-in-the-middle (MitM) attacks. Developers should enforce App Transport Security (ATS), which requires secure connections and provides a baseline for safe data transmission. Certificate pinning adds an extra layer of security by ensuring the app only trusts specific certificates, reducing the risk of connecting to malicious servers. Additionally, sensitive data should always be validated and sanitized before being sent or received to prevent injection attacks. By prioritizing secure network communication, developers can protect user information and maintain the integrity of app-server interactions.
Implementing SSL/TLS for Data Transmission
SSL/TLS ensures secure data transmission between iOS apps and backend servers. TLS provides encryption, authentication, and data integrity, preventing unauthorized access or tampering during communication. Developers should configure servers to use strong cipher suites and ensure proper certificate management to avoid vulnerabilities. To enhance security further, integrating white-box encryption can protect cryptographic keys within the app itself, even if it is reverse-engineered. Unlike traditional encryption, white-box encryption embeds key material to prevent its extraction, ensuring that sensitive data remains protected, even in compromised environments. By combining SSL/TLS with white-box encryption, developers can robustly defend against external threats, like MitM attacks, and internal risks, such as key exposure.
Protecting User Data in iOS Apps
Handling Sensitive Data Responsibly
Protecting sensitive user data is a cornerstone of iOS app security. Developers must adopt practices that minimize data exposure and ensure confidentiality, integrity, and availability. Sensitive information, such as personal identifiers, payment details, or authentication tokens, should never be stored in plain text. Instead, leverage secure storage solutions like the iOS Keychain or use file protection classes to encrypt data at rest. Data minimization principles should also be applied, collecting and storing only the data necessary for the app’s functionality. Proper access controls must be enforced, ensuring that sensitive data is accessible only to authenticated and authorized users. Furthermore, developers should ensure that all data transmitted over networks is encrypted using protocols like TLS. Responsibly handling sensitive data protects users and helps businesses maintain compliance with privacy regulations and build trust with their audience.
Implementing Data Minimization and Anonymization Techniques
Data minimization and anonymization are essential practices for reducing risk and ensuring user privacy in iOS applications. By adhering to data minimization principles, developers collect and retain only the data necessary to fulfill the app’s functionality, limiting exposure in the event of a breach.
Practice | Benefits |
---|---|
Anonymization Techniques | Enhances security by removing or obfuscating personally identifiable information (PII), ensuring that stored or transmitted data cannot be traced back to an individual. |
Tokenization, Hashing, and Encryption | Anonymizes sensitive data like user IDs or payment details while maintaining usability for key app functions. |
Automated Processes | Regularly review and purge unnecessary or outdated data. |
These practices safeguard user information and align with privacy regulations like GDPR and CCPA, fostering greater user trust and regulatory compliance.
Secure Storage Solutions
Leveraging Keychain Services
The iOS Keychain is a robust and secure storage solution designed specifically for sensitive data such as passwords, encryption keys, and authentication tokens. By leveraging Keychain Services, developers can ensure critical information is securely stored in an encrypted, hardware-backed environment. The Keychain automatically enforces access controls, restricting data access to the app or apps that created it and optionally requiring user authentication, such as Face ID or Touch ID. Furthermore, Keychain data is protected even if the device is compromised, thanks to its integration with iOS’s overall security architecture. Developers can access Keychain functionality using straightforward APIs, enabling seamless integration without sacrificing security. By adopting Keychain Services, iOS apps can securely manage sensitive user data while minimizing risks associated with insecure storage.
Protecting User Preferences and Data Files
Ensuring the security of user preferences and data files is critical for maintaining trust and preventing unauthorized access. On iOS, developers can utilize File Protection Classes to secure data files stored in the app’s sandbox. These classes leverage the device’s encryption capabilities, restricting access to files based on the app’s state, such as when the device is locked. For user preferences stored in NSUserDefaults, sensitive information should never be saved in plain text; instead, encrypt the data before storage or opt for secure alternatives like the Keychain for highly sensitive data. Additionally, developers should avoid storing sensitive files in easily accessible directories and ensure files are sanitized of any personally identifiable information when no longer needed. Implementing these measures ensures that user preferences and data remain protected, even when the device is lost, stolen, or compromised.
App Store Deployment Security Measures
App Review and Approval Process
The App Store review and approval process is a critical step in ensuring the security and integrity of iOS applications. Apple’s rigorous review standards are designed to identify and reject apps with security flaws, malicious behavior, or non-compliance with privacy policies. Developers should thoroughly test their apps for vulnerabilities, remove unnecessary permissions, and ensure compliance with Apple’s guidelines before submission. Incorporating secure coding practices, avoiding hardcoded sensitive data, and ensuring robust encryption is essential to passing this review. The review process also helps protect users by minimizing the likelihood of malicious or poorly secured apps being distributed, reinforcing the trust and reliability of the App Store ecosystem.
Monitoring and Responding to Security Incidents
Even after an app has been approved and deployed on the App Store, maintaining security is an ongoing responsibility. Developers should monitor for security incidents by integrating analytics and crash reporting tools to identify potential vulnerabilities or suspicious behavior. Swiftly addressing issues through patches or updates is essential to mitigate risks and protect users. Apple facilitates rapid responses with features like expedited app updates, allowing developers to deploy fixes quickly. Additionally, establishing a clear incident response plan, including communication strategies and user notification processes, ensures a proactive approach to managing security threats. Continuous monitoring and prompt action are key to sustaining user trust and app integrity in the dynamic mobile threat landscape.
Tools and Resources for iOS App Security
Recommended Security Libraries and Frameworks
Incorporating trusted security libraries and frameworks is a vital step for enhancing the security of iOS applications.
Library or Framework | Description |
---|---|
CryptoSwift | Provides easy-to-use implementations of cryptographic algorithms. |
OpenSSL | A reliable choice for advanced cryptographic needs. |
Alamofire | Supports HTTPS and TLS configurations for secure networking. |
TrustKit | Simplifies certificate pinning to prevent man-in-the-middle attacks. |
Firebase Authentication SDK | Offers robust tools for implementing secure user authentication. |
Parsec | Assist with encrypted data storage. |
By leveraging these libraries and frameworks, developers can implement advanced security measures efficiently and confidently.
Utilizing Apple’s Security Tools and Documentation
Apple provides a wealth of security tools and documentation to help developers create secure iOS apps. The Security framework includes APIs for cryptographic operations, secure storage, and key management, while Keychain Services offer a safe environment for sensitive data like passwords and tokens. Apple’s App Transport Security (ATS) ensures secure network communications by enforcing HTTPS. For best practices and detailed guidance, developers can refer to Apple’s official documentation and resources, such as the iOS Security Guide and Developer Forums. Regularly reviewing updates to Apple’s frameworks and guidelines ensures apps remain compliant with the latest security standards, empowering developers to stay ahead of evolving threats.

Application Security for Mobile: iOS
Explore
What's New In The World of Digital.ai
Announcing Quick Protect Agent: MASVS-Aligned Protections, Now Easier Than Ever
Easily apply OWASP MASVS-aligned protections to your mobile apps—no coding needed. Quick Protect Agent delivers enterprise-grade security in minutes.
“Think Like a Hacker” Webinar Recap: How AI is Reshaping App Security
Discover how generative AI is reshaping app security—empowering both developers and hackers. Learn key strategies to defend against AI-powered threats.
The Encryption Mandate: A Deep Dive into Securing Data in 2025
Discover how white-box cryptography and advanced encryption help enterprises secure sensitive data, meet compliance, and stay ahead of cybersecurity threats.