Table of Contents
Related Blogs
Overview of Client-Side Scripting
Client-side scripting plays a fundamental role in modern web development by enhancing user interactions and improving the overall experience of websites and applications. However, with these advantages come inherent security risks that need careful attention to prevent exploitation by malicious actors.
Definition and Examples
Client-side scripting refers to code executed directly in a user’s browser, rather than on the web server. It creates dynamic content, responds to user inputs, and renders interactive features. Unlike server-side code, which stays hidden from the user, client-side scripts are fully exposed, making them a potential target for threat actors.
Common examples of client-side scripting include form validations, animations, dynamic page updates without a full reload, and interactive maps. For instance, when a user fills out an online form and receives immediate feedback on errors (e.g., a missing required field), that’s the work of client-side scripting.
Commonly Used Client-Side Languages
Several languages are used to implement client-side scripts, but the most prominent ones include:
- JavaScript: By far the most widely used client-side scripting language, JavaScript enables interactive elements like dropdown menus, form submissions, and multimedia embeds.
- TypeScript: A superset of JavaScript, TypeScript adds static types, making it easier to catch errors during development while still compiling to JavaScript for browser compatibility.
- HTML & CSS: While not programming languages, both HTML and CSS play crucial roles in structuring and styling the content rendered by client-side scripts.
- Dart: Often used in conjunction with the Flutter framework for creating web and mobile applications.
- VBScript: Once popular in older versions of Internet Explorer, though largely deprecated now.
Each of these languages contributes to a web page’s functionality but must be secured to prevent exploitation.
Security Issues in Client-Side Scripting
Because client-side scripts run within the user’s browser, they are fully visible and modifiable, which opens several potential security vulnerabilities:
- Cross-Site Scripting (XSS): A common attack where malicious scripts are injected into trusted websites, allowing attackers to execute code in users’ browsers.
- Cross-Site Request Forgery (CSRF): Exploits a user’s authenticated session to perform unauthorized actions on a web application.
- Sensitive Data Exposure: Since client-side scripts can interact with sensitive data (e.g., tokens, user credentials), attackers may exploit poorly protected scripts to access this information.
- Reverse Engineering: Because client-side scripts are readily accessible, attackers can analyze and manipulate the code to discover vulnerabilities or extract valuable information about the underlying system.
These risks highlight the importance of applying robust security measures when implementing client-side scripting.
Best Practices for Secure Client-Side Scripting
Input Validation and Sanitization
Proper input validation and sanitization are critical for preventing attacks like Cross-Site Scripting (XSS) and SQL injection. Input validation ensures that the data users provide matches the expected format (e.g., numbers, email addresses), while sanitization removes any potentially harmful characters or scripts from input fields. Always validate inputs both on the client side for immediate feedback and on the server side for comprehensive security. Implementing strong validation and sanitization routines helps mitigate the risk of malicious data compromising the integrity of your application.
Use of HTTPS
Using HTTPS is essential for securing client-server communication by encrypting the data exchanged between the user’s browser and your server. HTTPS protects sensitive information such as login credentials, payment details, and session tokens from being intercepted by attackers through man-in-the-middle (MITM) attacks. Implementing HTTPS across your entire site ensures that all client-side scripts, resources, and interactions are encrypted, boosting security and user trust. Make sure to enforce HTTPS through HTTP Strict Transport Security (HSTS) headers for maximum protection.
Content Security Policy (CSP)
A Content Security Policy (CSP) helps prevent attacks like Cross-Site Scripting (XSS) by controlling the sources from which a browser can load resources such as scripts, styles, and images. By defining a CSP, you can restrict where scripts and content can be loaded from, effectively reducing the risk of malicious content execution. For example, you can allow scripts only from trusted domains while blocking inline scripts. Implementing a robust CSP is a powerful way to mitigate the risk of client-side vulnerabilities and ensure that your application only executes approved content.
Avoiding Inline Scripts
Avoiding inline scripts is a critical best practice for securing client-side code. Inline scripts are more vulnerable to attacks like Cross-Site Scripting (XSS), as attackers can easily inject or modify them. Instead of embedding JavaScript directly in HTML, place scripts in external files and reference them through secure sources. This enhances security by enabling the use of Content Security Policy (CSP), improves code maintainability, and reduces the attack surface for client-side vulnerabilities.
Proper Error Handling
Proper error handling is essential for preventing the exposure of sensitive information to users or attackers. Client-side errors, when not managed securely, can reveal details about the application’s inner workings, potentially aiding attackers in discovering vulnerabilities. Instead of displaying detailed error messages, ensure that error messages are generic and do not disclose stack traces or system-specific information. Additionally, log errors on the server side for further investigation, while providing users with a simple, user-friendly message that doesn’t compromise security.
Obfuscation
Obfuscation is the process of deliberately making your client-side code harder to understand in order to prevent reverse engineering and unauthorized tampering. By transforming readable code into a more cryptic format, obfuscation adds a layer of security that deters attackers from easily analyzing or exploiting your scripts. Combining obfuscation with techniques like minification and encryption frustrates efforts to reverse-engineer your application, making it harder for threat actors to uncover vulnerabilities or sensitive logic.
Anti-Tamper
Anti-tamper techniques detect and prevent unauthorized modifications to your client-side code. These measures include integrity checks that verify whether scripts have been altered or injected with malicious code. If tampering is detected, anti-tamper mechanisms trigger responses, such as disabling the application, alerting the server, or logging suspicious activity. By implementing anti-tamper solutions, you can add an additional layer of security that makes it difficult for attackers to modify or manipulate your code to exploit vulnerabilities and exposed logic.
Protecting Sensitive Data
Encryption Techniques
Encrypting sensitive data is crucial for protecting it from unauthorized access during storage and transmission. Common encryption techniques include symmetric and asymmetric encryption, both of which rely on cryptographic keys to secure data. In addition to these, White Box Cryptography is specifically designed to safeguard encryption keys, even when stored in potentially insecure environments such as client-side applications. By embedding the keys within complex, obfuscated operations, White Box Cryptography prevents attackers from extracting them, making it an essential technique for securing sensitive data in client-side code.
Secure Transmission
Ensuring secure data transmission between the client and server is critical to preventing interception and tampering. Transport Layer Security (TLS) should always be used to encrypt data in transit, protecting it from man-in-the-middle (MITM) attacks. This ensures that sensitive information, such as login credentials and financial data, remains confidential and integral as it travels over the network. Additionally, using secure protocols like HTTPS with proper certificates helps maintain data integrity during transmission.
Token-Based Authentication
Token-based authentication is a secure method for verifying user identities in client-server interactions. Instead of storing sensitive credentials, the server issues a token (such as a JSON Web Token or JWT) after successful authentication, which is then used for subsequent requests. These tokens are stateless and can be securely transmitted via HTTPS. Token-based authentication helps mitigate risks such as session hijacking and replay attacks by ensuring that only valid tokens are accepted for authorized actions, enhancing the security of client-side applications.
Browser Security Features
Same-Origin Policy
The Same-Origin Policy (SOP) is a fundamental browser security feature that restricts how scripts on one web page can interact with resources from another origin (i.e., domain, protocol, or port). This policy prevents malicious websites from accessing sensitive data or performing unauthorized actions on another site. By enforcing SOP, browsers help protect users from attacks such as Cross-Site Request Forgery (CSRF) and data theft, ensuring that web pages can only interact with resources from the same origin unless explicitly permitted through mechanisms like Cross-Origin Resource Sharing (CORS).
Secure Cookies
Secure cookies are a critical feature for protecting sensitive data stored in the user’s browser. Marking a cookie with the `Secure` attribute, ensures the cookie is only transmitted over HTTPS, preventing attackers from intercepting it in transit. Additionally, using the `HttpOnly` attribute prevents client-side scripts from accessing cookies, reducing the risk of Cross-Site Scripting (XSS) attacks. Properly configuring secure cookies helps safeguard session tokens and other sensitive data, ensuring they remain confidential and protected from tampering.
HTTP Headers for Security (e.g., HSTS, X-Content-Type-Options)
HTTP security headers provide an additional layer of protection for client-side applications by instructing browsers on how to handle certain types of requests. HTTP Strict Transport Security (HSTS) enforces the use of HTTPS, ensuring that all communications occur over a secure channel. X-Content-Type-Options prevents browsers from interpreting files as a different MIME type than specified, helping to block certain attacks like drive-by downloads or Cross-Site Scripting (XSS). Implementing these headers strengthens the overall security posture of your web application and mitigates common attack vectors.
Tools and Libraries for Enhancing Security
Security Linters and Scanners
Security linters and scanners are essential tools for identifying potential vulnerabilities in your client-side code before it goes into production. Linters, like ESLint with security plugins, help detect insecure coding practices such as unsanitized inputs or unsafe use of APIs. S On the other hand, security scanners analyze your application for known vulnerabilities, misconfigurations, or outdated libraries. Integrating these tools into your development workflow lets you catch and address security issues early, ensuring a more secure client-side environment.
Frameworks and Libraries (e.g., Angular, React) Security Features
Modern frameworks like Angular and React offer built-in security features to help developers safeguard client-side applications. Angular provides mechanisms like automatic input sanitization to prevent Cross-Site Scripting (XSS) attacks, while React encourages secure coding practices by default, such as preventing direct DOM manipulation. Both frameworks emphasize the importance of using secure practices, like proper state management and avoiding unsafe API usage, to help reduce common vulnerabilities in client-side code.
Using Application Hardening Tools
Application hardening tools, such as Runtime Application Self-Protection (RASP), obfuscation, anti-tamper, and client-side app threat monitoring, provide robust defense mechanisms against attacks targeting client-side applications. RASP detects and blocks threats in real time, safeguarding applications during execution. Obfuscation makes code difficult to understand and reverse-engineer, while anti-tamper mechanisms ensure that any unauthorized changes to the code are detected and mitigated. Client-side app threat monitoring continuously tracks suspicious activity, providing visibility into potential attacks. Together, these tools significantly increase the security of your client-side applications, making them much harder to compromise.
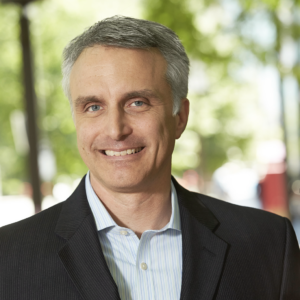
Application Security for Web
Explore
What's New In The World of Digital.ai
Announcing Quick Protect Agent: MASVS-Aligned Protections, Now Easier Than Ever
Easily apply OWASP MASVS-aligned protections to your mobile apps—no coding needed. Quick Protect Agent delivers enterprise-grade security in minutes.
“Think Like a Hacker” Webinar Recap: How AI is Reshaping App Security
Discover how generative AI is reshaping app security—empowering both developers and hackers. Learn key strategies to defend against AI-powered threats.
The Encryption Mandate: A Deep Dive into Securing Data in 2025
Discover how white-box cryptography and advanced encryption help enterprises secure sensitive data, meet compliance, and stay ahead of cybersecurity threats.